# 背景
在写浏览器调用摄像头进行人脸识别的时候,
需要获取关键帧进行扫描解析,
刚好,写了一个获取摄像头图像的Demo,
放上来一起分享
# 运行原理
过程比较简单,
我就直接简单概述一下
流程:
- 利用 WebRTC 调用摄像头
- 将 WebRTC 获取到的 视频流 传送到 video 标签
- 利用 requestAnimationFrame 方法,定时将画面同步到 canvas 标签
- 点击按钮的时候,直接从 canvas 元素获取当前画面的 base64 数据,利用方法 toDataURL
- 将 base64 数据展示到 img 标签
# 代码
我直接将代码文件放到我群里: 492781269
可以加群下载
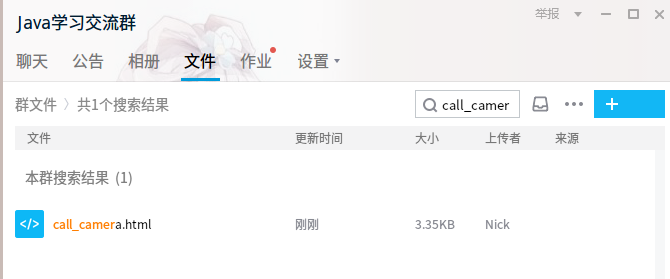
下面还是贴一下完整的代码吧:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>调用摄像头截图拍照(WebRtc-video-canvas-img)</title> </head> <body> <div id="videoBox"> <video src="" id="myVideo" autoplay="autoplay"></video> <canvas id="output" style="display: none"></canvas> <button id="myButton">截图(拍照)</button> <img id="myImg" src="" alt=""> </div>
<script> const myVideo = document.querySelector("#myVideo"); const myCanvas = document.querySelector("#output"); const ctx = myCanvas.getContext("2d"); const myButton = document.querySelector("#myButton"); const myImg = document.querySelector("#myImg");
function getCanvasBase64() { return myCanvas.toDataURL("image/jpeg", 1); }
function canvasFrame() { ctx.drawImage(myVideo, 0, 0, myCanvas.width, myCanvas.height);
requestAnimationFrame(canvasFrame); }
function setupCamera() { let exArray = []; navigator.getUserMedia = navigator.getUserMedia || navigator.webkitGetUserMedia || navigator.mozGetUserMedia || navigator.msGetUserMedia; navigator.mediaDevices.enumerateDevices() .then(function (sourceInfos) { for (var i = 0; i < sourceInfos.length; ++i) { if (sourceInfos[i].kind == 'videoinput') { exArray.push(sourceInfos[i].deviceId); } } }) .then(() => { let deviceId = exArray[exArray.length - 1];
navigator.mediaDevices.getUserMedia({ audio: false, video: { deviceId: deviceId } }) .then(stream => { myVideo.srcObject = stream; myVideo.onloadedmetadata = () => { myVideo.width = myVideo.offsetWidth; myVideo.height = myVideo.offsetHeight; myCanvas.width = myVideo.width; myCanvas.height = myVideo.height; canvasFrame(); }; }) .catch(err => { console.log }); }); }
myButton.addEventListener('click', () => { let imageBase64 = getCanvasBase64(); myImg.src = imageBase64; });
window.addEventListener('load', () => { setupCamera(); });
</script> </body> </html>
|
PS:
如有错误,还请多多指出来~
– Nick
– 2019/07/22